
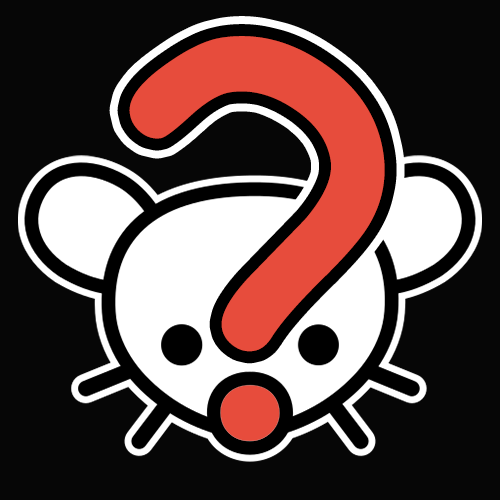
Exactly who I was thinking of. I actually really appreciate that they evolved their music, instead of just doing the same things for 15 years.
Exactly who I was thinking of. I actually really appreciate that they evolved their music, instead of just doing the same things for 15 years.
The XZ exploit was found because some dude was investigating performance issues in a system, and noticed an unusual amount of time being spent in SSH processing, IIRC.
So, you’re a tech nerd who wants an addictive game?
Factorio.
Also Satisfactory, but I’m not sure how well it runs on Linux. Fairly sure Factorio will run on just about anything
Windows 11 has ads NOW, in the enterprise install I’m provided at work.
MLB 66
For work, I have 2 monitors, and my docked laptop. The main two monitors are hugely beneficial for software development, as I can reference design docs or requirements while writing code, or I can have the debugger running on one screen, while the app runs on the other.
The laptop screen is where Teams and Outlook sit, so I can glance over at messages from the team, and maybe respond, without having to swap around any of my workspace.
I’ll take this over the more “classic” styles, when people seed to believe they were paying the compiler by the character.
You mention Bally and baseball, mlb66 was my go-to last year.
Not really, no.
Generally speaking, fault protection schemes need only account for one fault at a time, unless you’re a really large business, or some other entity with extra-stringent data protection requirements.
RAID protects against drive failure faults. Backups protect against drive failure faults as well, but also things like accidental deletions or overwrites of data.
In order for RAID on backups to make sense, when you already have RAID on your main storage, you’d have to consider drive failures and other data loss to be likely to occur simultaneously. I.E. RAID on your backups only protects you from drive failure occurring WHILE you’re trying to restore a backup. Or maybe more generally, WHILE that backup is in use, say, if you have a legal requirement that you must keep a history of all your data for X years or something (I would argue data like this shouldn’t be classified as backups, though).
The point is that everyone does have them, but only rarely are they visible to the human eye.
Damn, actual personal growth being displayed on the internet? Such a rare thing I find myself wondering it wasn’t all staged. How messed up is that?
Also, how messed up is it that it worked, cause I’mm’a go watch all of these.
The “base password” concept isn’t completely crazy, I’ve got a friend who claims to have a system like this, to keep all his passwords in memory.
The key, though, is that the modification to the base is NOT just “usbank” or “facebook” or whatever. If your system for modifying the base is too simple, then if one of the sites you do this for is breached, and its passwords exposed, you can bet that attackers that get ahold of those are smart enough to search for “usbank” and “facebook” and other variations, to figure out what your base password is, and take that and apply it on as many other sites as they can, and may well breach your other accounts.
Can I get an Oracle version?
There was a Payday 3?
Dark & Stormy is my favorite, from what I’ve tried
Why the hell is a professional tech business not relying almost-exclusively in ethernet, anyway?
Unless you’re doing something crazy, like super-long runs (like, across a whole building) get the cheapest thing you can find that’s rated for the minimum spec you need (like, if you want 4k at 120fps or whatever, lookup which HDMI version is needed for that, and buy at least that). That means do NOT buy at any brick-and-mortar store, their markups are borderline illegal.
I’ll drop another recommendation for monoprice.com.
Discord gained popularity and maintains it, in spite of the many reasons to avoid it, because of usability and feature richness. Slack, Teams, Matrix, Telegram, they are miles ahead of everyone else in the live-chat space, when it comes to user experience.
This was an interesting article about some tech I’ve never heard of before, but it has little to nothing to do with Discord’s overall success.
Shit shit shit, I just remembered I haven’t attended English class all semester.
Shit shit shit, I can’t remember my locker combination, and I can’t find the orientation sheet that has it, also I can’t find my class schedule, I have no idea what class I’m supposed to be in right now.
Plus a few other variations. All High School. I dunno why the focus on High School, I’m 34. I get one of these once or twice a month.